List
<List>
provides us a layout to display the page. It does not contain any logic but adds extra functionalities like a create button or giving the page titles.
We will show what <List>
does using properties with examples.
Properties
canCreate
and createButtonProps
canCreate
allows us to add the create button inside the <List>
component. If resource is passed a create component, refine adds the create button by default. If you want to customize this button you can use createButtonProps
property like the code below.
Create button redirects to the create page of the resource according to the value it reads from the URL.
import { List, usePermissions } from "@pankod/refine";
export const ListPage: React.FC = () => {
const { data } = usePermissions<string>();
return (
<List
canCreate={data === "admin"}
createButtonProps={{ size: "small" }}
>
...
</List>
);
};
Refer to the usePermission
documentation for detailed usage. →
title
It allows adding a title for the <List>
component. if you don't pass title props, it uses plural from of resource name by default.
import { List } from "@pankod/refine";
export const ListPage: React.FC = () => {
return <List title="Custom Title">...</List>;
};
pageHeaderProps
<List>
uses ant-design <PageHeader>
components so you can customize with the props of pageHeaderProps
.
Refer to the <PageHeader>
documentation for detailed usage. →
import { List } from "@pankod/refine";
export const ListPage: React.FC = () => {
return (
<List
pageHeaderProps={{
onBack: () => console.log("clicked"),
subTitle: "Subtitle",
}}
>
...
</List>
);
};
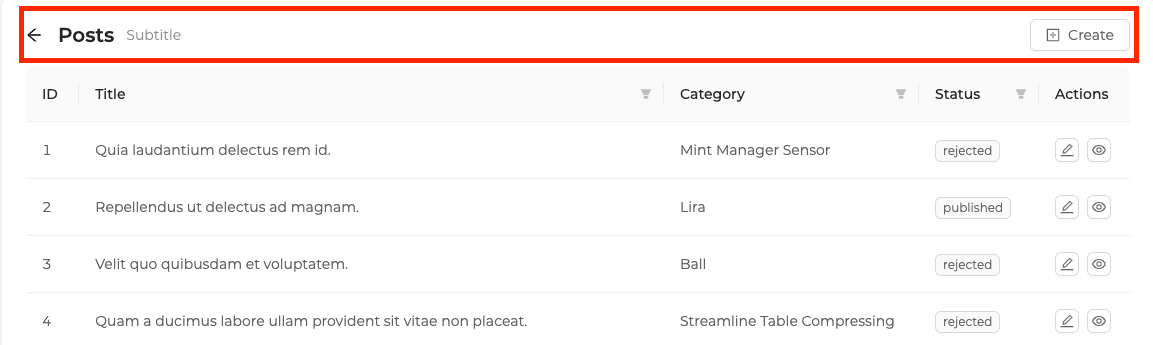
resource
<List>
component reads the resource
information from the route by default. This default behavior will not work on custom pages. If you want to use the <List>
component in a custom page, you can use the resource
prop.
Refer to the custom pages documentation for detailed usage. →
import { Refine, List } from "@pankod/refine";
import routerProvider from "@pankod/refine-react-router";
import dataProvider from "@pankod/refine-simple-rest";
const CustomPage = () => {
return <List resource="posts">...</List>;
};
export const App: React.FC = () => {
return (
<Refine
routerProvider={{
...routerProvider,
routes: [
{
exact: true,
component: CustomPage,
path: "/custom",
},
]
}}
dataProvider={dataProvider("https://api.fake-rest.refine.dev/")}
resources={[{ name: "posts" }]}
/>
);
};
API Reference
Properties
Property | Description | Type | Default |
---|---|---|---|
canCreate | Adds create button | boolean | If the resource is passed a create component, true else false |
createButtonProps | Adds props for create button | ButtonProps & { resourceName: string } | <CreateButton /> |
title | Adds title | string | Plural of resource.name |
pageHeaderProps | Passes properties for <PageHeader> | PageHeaderProps | { ghost: false, title, extra: <CreateButton /> } |
resource | Resource name for API data interactions | string | Resource name that it reads from the url. |