Create
<CreateButton>
uses Ant Design's <Button>
component. It uses the create
method from useNavigation
under the hood. It can be useful to redirect the app to the create page route of resource.
Usage
import {
CreateButton,
List,
Table,
useTable,
} from "@pankod/refine";
export const PostList: React.FC = () => {
const { tableProps } = useTable<IPost>();
return (
<List pageHeaderProps={{ extra: <CreateButton /> }}>
<Table {...tableProps} rowKey="id">
<Table.Column dataIndex="id" title="ID" />
<Table.Column dataIndex="title" title="Title" />
</Table>
</List>
);
};
interface IPost {
id: string;
title: string;
}
Will look like this:
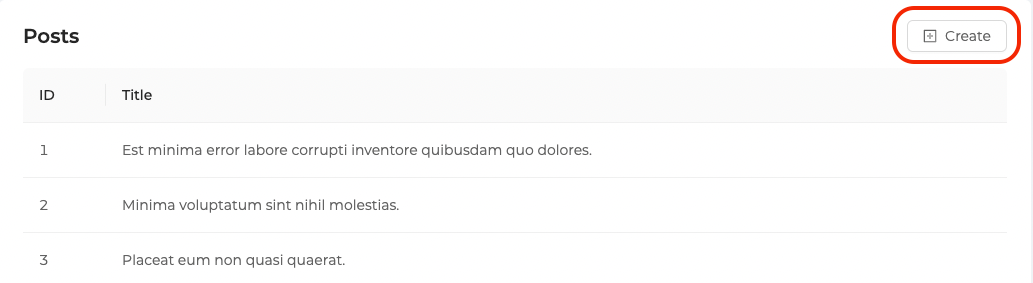
Properties
resourceName
It is used to redirect the app to the /create
endpoint of the given resource name. By default, the app redirects to a URL with /create
defined by the name property of resource object.
import { CreateButton } from "@pankod/refine";
export const MyCreateComponent = () => {
return <CreateButton resourceName="posts" />;
};
Clicking the button will trigger the create
method of useNavigation
and then redirect to /posts/create
.
hideText
It is used to show and not show the text of the button. When true
, only the button icon is visible.
import { CreateButton } from "@pankod/refine";
export const MyCreateComponent = () => {
return <CreateButton hideText />;
};
ignoreAccessControlProvider
It is used to skip access control for the button so that it doesn't check for access control. This is relevant only when an accessControlProvider
is provided to <Refine/>
import { CreateButton } from "@pankod/refine";
export const MyListComponent = () => {
return <CreateButton ignoreAccessControlProvider />;
};
API Reference
Properties
Property | Description | Type | Default |
---|---|---|---|
props | Ant Design button props | ButtonProps & { resourceName?: string; hideText?: boolean; } | |
resourceName | Determines which resource to use for redirection | string | Resource name that it reads from route |
hideText | Allows to hide button text | boolean | false |
ignoreAccessControlProvider | Skip access control | boolean | false |
children | Sets the button text | ReactNode | "Create" |
icon | Sets the icon component of button | ReactNode | <PlusSquareOutlined /> |
onClick | Sets the handler to handle click event | (event) => void | Triggers navigation for redirect to the create page of resource |