Edit
<EditButton>
uses Ant Design's <Button>
component. It uses the edit
method from useNavigation
under the hood. It can be useful when redirecting the app to the edit page with the record id route of resource.
Usage
import {
List,
Table,
useTable,
EditButton,
} from "@pankod/refine";
export const PostList: React.FC = () => {
const { tableProps } = useTable<IPost>();
return (
<List>
<Table {...tableProps} rowKey="id">
<Table.Column dataIndex="id" title="ID" />
<Table.Column dataIndex="title" title="Title" />
<Table.Column<IPost>
title="Actions"
dataIndex="actions"
key="actions"
render={(_, record) => (
<EditButton size="small" recordItemId={record.id} />
)}
/>
</Table>
</List>
);
};
interface IPost {
id: string;
title: string;
}
Will look like this:
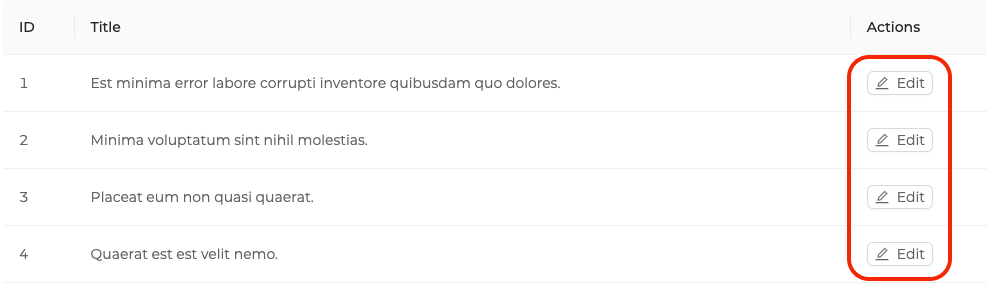
Properties
recordItemId
recordItemId
is used to append the record id to the end of the route path.
import { EditButton } from "@pankod/refine";
export const MyEditComponent = () => {
return <EditButton resourceName="posts" recordItemId="1" />;
};
Clicking the button will trigger the edit
method of useNavigation
and then redirect the app to /posts/edit/1
.
<EditButton>
component reads the id information from the route by default.
resourceName
Redirection endpoint(resourceName/edit
) is defined by resourceName
property. By default, <EditButton>
uses name
property of the resource object as an endpoint to redirect after clicking.
import { EditButton } from "@pankod/refine";
export const MyEditComponent = () => {
return <EditButton resourceName="categories" recordItemId="2" />;
};
Clicking the button will trigger the edit
method of useNavigation
and then redirect the app to /categories/edit/2
.
hideText
It is used to show and not show the text of the button. When true
, only the button icon is visible.
import { EditButton } from "@pankod/refine";
export const MyEditComponent = () => {
return <EditButton hideText />;
};
ignoreAccessControlProvider
It is used to skip access control for the button so that it doesn't check for access control. This is relevant only when an accessControlProvider
is provided to <Refine/>
import { EditButton } from "@pankod/refine";
export const MyListComponent = () => {
return <EditButton ignoreAccessControlProvider />;
};
API Reference
Properties
Property | Description | Type | Default |
---|---|---|---|
props | Ant Design button properties | ButtonProps & { resourceName?: string; recordItemId?: string; hideText?: boolean; } | |
resourceName | Determines which resource to use for redirection | string | Resource name that it reads from route |
recordItemId | Adds id to the end of the URL | string | Record id that it reads from route |
hideText | Allows to hide button text | boolean | false |
ignoreAccessControlProvider | Skip access control | boolean | false |
children | Sets the button text | ReactNode | "Edit" |
icon | Sets the icon component of button | ReactNode | <EditOutlined /> |
onClick | Sets the handler to handle click event | (event) => void | Triggers navigation for redirection to the edit page of resource |