Show
<ShowButton>
uses Ant Design's <Button>
component. It uses the show
method from useNavigation
under the hood. It can be useful when redirecting the app to the show page with the record id route of resource.
Usage
import {
List,
Table,
useTable,
ShowButton,
} from "@pankod/refine";
export const PostList: React.FC = () => {
const { tableProps } = useTable<IPost>();
return (
<List>
<Table {...tableProps} rowKey="id">
<Table.Column dataIndex="id" title="ID" />
<Table.Column dataIndex="title" title="Title" />
<Table.Column<IPost>
title="Actions"
dataIndex="actions"
render={(_, record) => (
<ShowButton size="small" recordItemId={record.id} />
)}
/>
</Table>
</List>
);
};
interface IPost {
id: string;
title: string;
}
Will look like this:
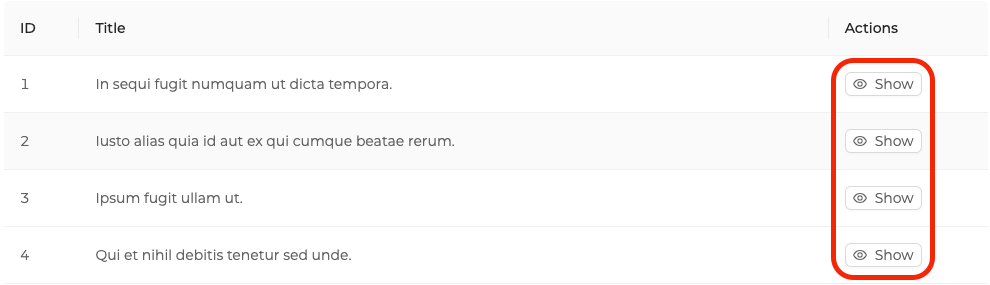
Properties
recordItemId
recordItemId
is used to append the record id to the end of the route path.
import { ShowButton } from "@pankod/refine";
export const MyShowComponent = () => {
return <ShowButton resourceName="posts" recordItemId="1" />;
};
Clicking the button will trigger the show
method of useNavigation
and then redirect the app to /posts/show/1
.
<ShowButton>
component reads the id information from the route by default.
resourceName
Redirection endpoint(resourceName/show
) is defined by resourceName
property. By default, <ShowButton>
uses name
property of the resource object as an endpoint to redirect after clicking.
import { ShowButton } from "@pankod/refine";
export const MyShowComponent = () => {
return <ShowButton resourceName="categories" recordItemId="2" />;
};
Clicking the button will trigger the show
method of useNavigation
and then redirect the app to /categories/show/2
.
hideText
It is used to show and not show the text of the button. When true
, only the button icon is visible.
import { ShowButton } from "@pankod/refine";
export const MyShowComponent = () => {
return <ShowButton hideText />;
};
ignoreAccessControlProvider
It is used to skip access control for the button so that it doesn't check for access control. This is relevant only when an accessControlProvider
is provided to <Refine/>
import { ListButton } from "@pankod/refine";
export const MyListComponent = () => {
return <ListButton ignoreAccessControlProvider />;
};
API Reference
Properties
Property | Description | Type | Default |
---|---|---|---|
props | Ant Design button properties | ButtonProps & { resourceName?: string; recordItemId?: string; hideText?: boolean; } | |
resourceName | Determines which resource to use for redirection | string | Resource name that it reads from route |
recordItemId | Adds id to the end of the URL | string | Record id that it reads from route |
hideText | Allows to hide button text | boolean | false |
ignoreAccessControlProvider | Skip access control | boolean | false |
children | Sets the button text | ReactNode | "Show" |
icon | Sets the icon component of button | ReactNode | <EyeOutlined /> |
onClick | Sets the handler to handle click event | (event) => void | Triggers navigation for redirection to the show page of resource |