useShow
useShow
hook allows you to fetch the desired record. It uses getOne
method as query function from the dataProvider that is passed to <Refine>
.
const { queryResult } = useShow();
When no property is given, it tries to read the resource
and id
information from the route.
Usage
First, we'll create a page to show the records. Then we'll use this page for the show property of the resource.
src/pages/posts/show.tsx
import { useShow, Show, Typography } from "@pankod/refine";
const { Title, Text } = Typography;
export const PostShow: React.FC = () => {
const { queryResult } = useShow<IPost>();
const { data, isLoading } = queryResult;
const record = data?.data;
return (
<Show isLoading={isLoading}>
<Title level={5}>Id</Title>
<Text>{record?.id}</Text>
<Title level={5}>Title</Title>
<Text>{record?.title}</Text>
</Show>
);
};
interface IPost {
id: string;
title: string;
}
We didn't give any property to useShow
because it can read resource
and id
information from the route.
src/App.tsx
import { Refine } from "@pankod/refine";
import routerProvider from "@pankod/refine-react-router";
import dataProvider from "@pankod/refine-json-server";
import { PostShow } from "./pages/posts";
export const App: React.FC = () => {
return (
<Refine
routerProvider={routerProvider}
dataProvider={dataProvider("https://api.fake-rest.refine.dev")}
resources={[{ name: "posts", show: PostShow }]}
/>
);
};
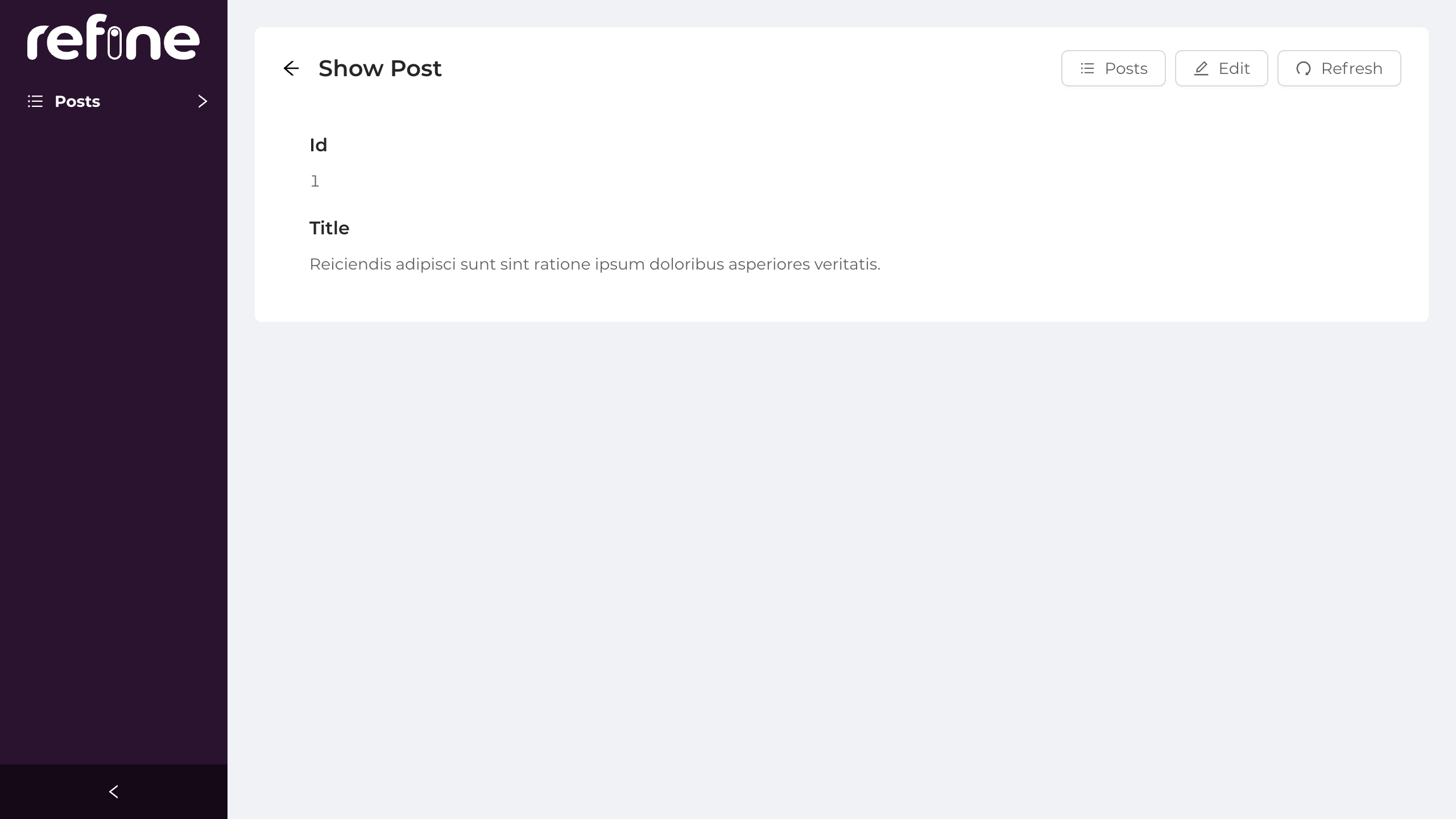
In the next example, we'll show how it is used for the modal.
Let's simply create a post list showing posts.
src/pages/posts/list.tsx
import { List, Table, useTable } from "@pankod/refine";
export const PostList: React.FC = () => {
const { tableProps } = useTable<IPost>();
return (
<List>
<Table {...tableProps} rowKey="id">
<Table.Column dataIndex="id" title="ID" />
<Table.Column dataIndex="title" title="Title" />
</Table>
</List>
);
};
Let's add our modal.
src/pages/posts/list.tsx
import {
List,
Table,
useTable,
Modal,
Show,
ShowButton,
Typography,
useShow,
} from "@pankod/refine";
const { Title, Text } = Typography;
export const PostList: React.FC = () => {
const [visible, setVisible] = useState(false);
const { tableProps } = useTable<IPost>();
const { queryResult, showId, setShowId } = useShow<IPost>();
const { data, isLoading } = queryResult;
const record = data?.data;
return (
<>
<List>
<Table {...tableProps} rowKey="id">
<Table.Column dataIndex="id" title="ID" />
<Table.Column dataIndex="title" title="Title" />
<Table.Column<IPost>
title="Actions"
dataIndex="actions"
render={(_, record) => (
<ShowButton
size="small"
recordItemId={record.id}
onClick={() => {
setShowId(record.id);
setVisible(true);
}}
/>
)}
/>
</Table>
</List>
<Modal visible={visible} onCancel={() => setVisible(false)}>
<Show isLoading={isLoading} recordItemId={showId}>
<Title level={5}>Id</Title>
<Text>{record?.id}</Text>
<Title level={5}>Title</Title>
<Text>{record?.title}</Text>
</Show>
</Modal>
</>
);
};
Finally, let's pass this page to the resources
as a list component.
src/App.tsx
import { Refine } from "@pankod/refine";
import routerProvider from "@pankod/refine-react-router";
import dataProvider from "@pankod/refine-json-server";
import { PostList } from "./pages/posts";
export const App: React.FC = () => {
return (
<Refine
routerProvider={routerProvider}
dataProvider={dataProvider("https://api.fake-rest.refine.dev")}
resources={[{ name: "posts", list: PostList }]}
/>
);
};
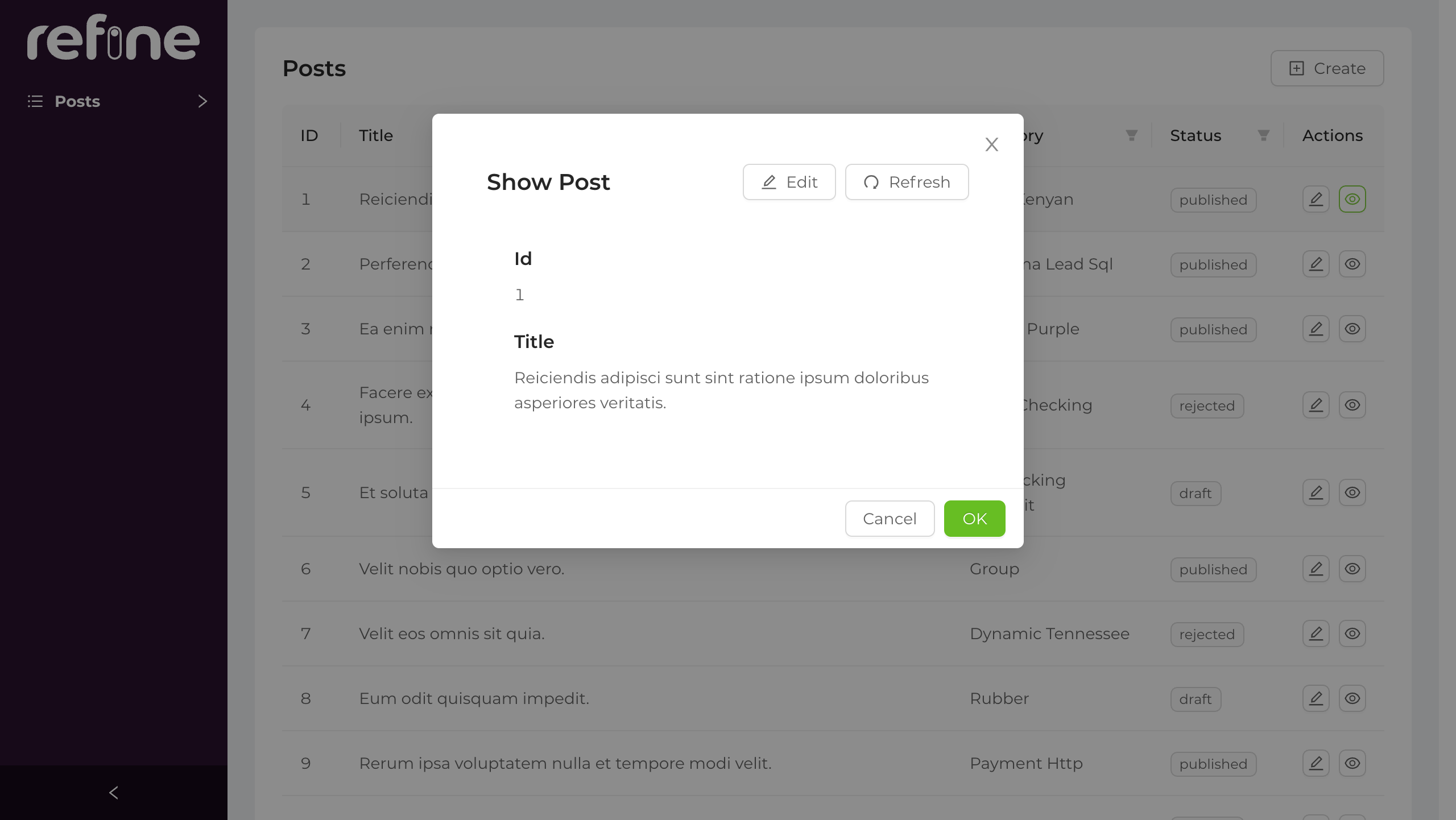
tip
To show data in the drawer, you can do it by simply replacing <Modal>
with <Drawer>
.
API Reference
Properties
Property | Description | Type | Default |
---|---|---|---|
resource | Resource name for API data interactions | string | Resource name that it reads from the url |
id | Record id for fetching | string | Id that it reads from the URL |
metaData | Metadata query for dataProvider | MetaDataQuery | {} |
liveMode | Whether to update data automatically ("auto" ) or not ("manual" ) if a related live event is received. The "off" value is used to avoid creating a subscription. | "auto" | "manual" | "off" | "off" |
liveParams | Params to pass to liveProvider 's subscribe method if liveMode is enabled. | { ids?: string[]; [key: string]: any; } | undefined |
onLiveEvent | Callback to handle all related live events of this hook. | (event: LiveEvent) => void | undefined |
Return values
Property | Description | Type |
---|---|---|
queryResult | Result of the query of a record | QueryObserverResult<{ data: TData }> |
showId | Record id | string |
setShowId | showId setter | Dispatch<SetStateAction< string | undefined>> |