useSimpleList
By using useSimpleList
you get props for your records from API in accordance with Ant Design <List>
component. All features such as pagination, sorting come out of the box.
Refer to Ant Design docs for <List>
component information →
Usage
Let's assume that the data we will show in the table comes from the endpoint as follows:
https://api.fake-rest.refine.dev/posts
[
{
"id": 182,
"title": "A aspernatur rerum molestiae.",
"content": "Natus molestias incidunt voluptatibus. Libero delectus facilis...",
"hit": 992123,
"category": {
"id": 1,
"title": "Navigating"
}
},
{
"id": 989,
"title": "A molestiae vel voluptatem enim.",
"content": "Voluptas consequatur quia beatae. Ipsa est qui culpa deleniti...",
"hit": 29876,
"category": {
"id": 2,
"title": "Empowering"
}
}
]
If we want to make a listing page where we show the title
, content
, hit
and category.title
values:
import {
PageHeader,
Typography,
useMany,
AntdList,
useSimpleList,
NumberField,
Space,
} from "@pankod/refine";
export const PostList: React.FC = () => {
const { Text } = Typography;
const { listProps } = useSimpleList<IPost>({
initialSorter: [
{
field: "id",
order: "asc",
},
],
pagination: {
pageSize: 6,
},
});
const categoryIds =
listProps?.dataSource?.map((item) => item.category.id) ?? [];
const { data } = useMany<ICategory>({
resource: "categories",
ids: categoryIds,
queryOptions: {
enabled: categoryIds.length > 0,
},
});
const renderItem = (item: IPost) => {
const { title, hit, content } = item;
const categoryTitle = data?.data.find(
(category: ICategory) => category.id === item.category.id,
)?.title;
return (
<AntdList.Item
actions={[
<Space key={item.id} direction="vertical" align="end">
<NumberField
value={hit}
options={{
// @ts-ignore
notation: "compact",
}}
/>
<Text>{categoryTitle}</Text>
</Space>,
]}
>
<AntdList.Item.Meta title={title} description={content} />
</AntdList.Item>
);
};
return (
<PageHeader ghost={false} title="Posts">
<AntdList {...listProps} renderItem={renderItem} />
</PageHeader>
);
};
interface IPost {
id: string;
title: string;
content: string;
hit: number;
category: ICategory;
}
interface ICategory {
id: string;
title: string;
}
tip
You can use AntdList.Item
and AntdList.Item.Meta
like <List>
component from Ant Design
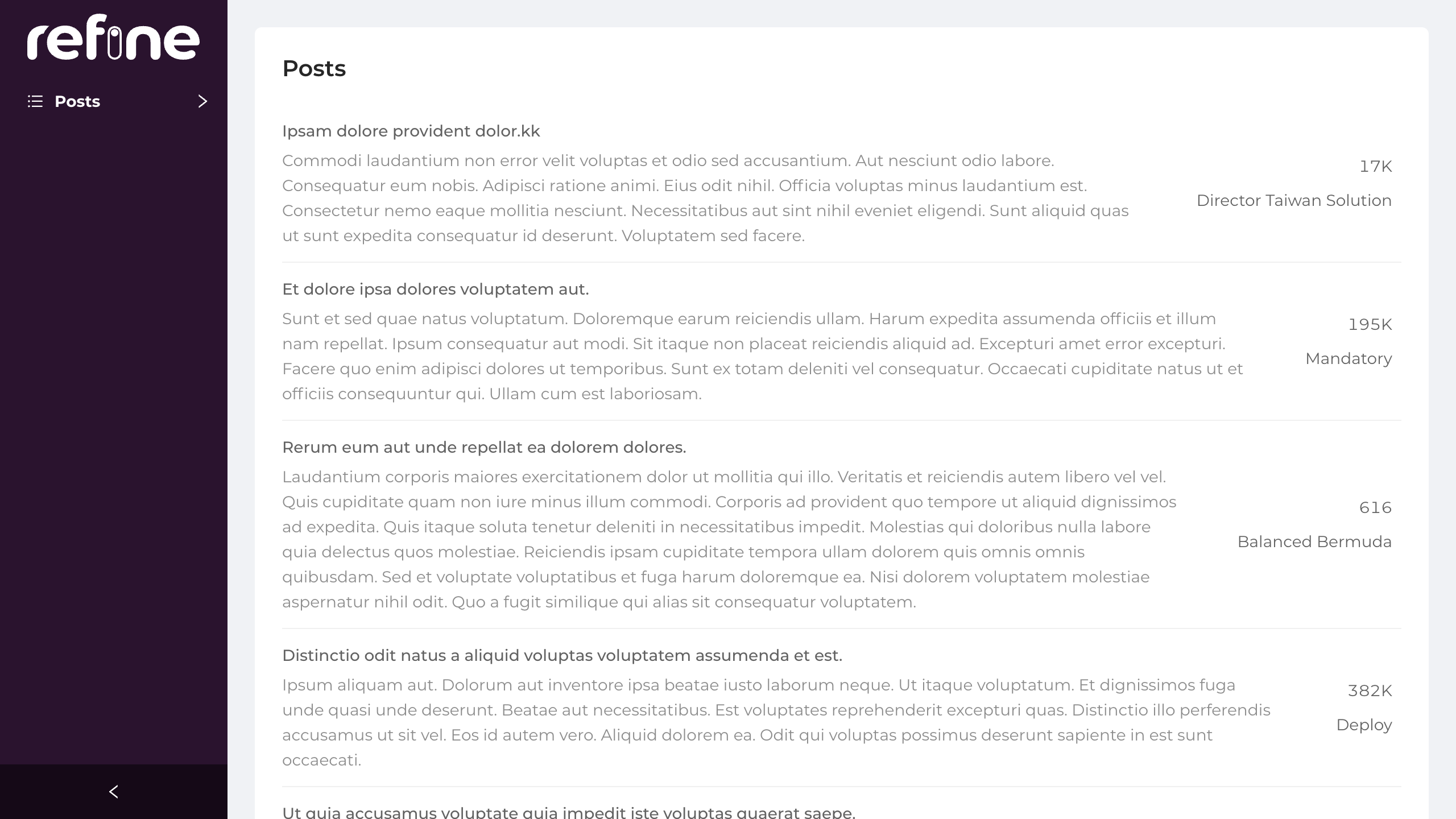
API
Properties
Key | Description | Type | Default |
---|---|---|---|
resource | The resource to list the data | string | undefined | Resource name that it reads from the url |
permanentFilter | Default and unchangeable filter | CrudFilters | [] |
initialSorter | Allows to sort records by speficified order and field | CrudSorting | |
initialFilter | Allows to filter records by speficified order and field | CrudFilters | |
listProps | Ant Design <List> props | listProps | |
syncWithLocation | Sortings, filters, page index and records shown per page are tracked by browser history | boolean | Value set in Refine. If a custom resource is given, it will be false |
onSearch | When the search form is submitted, it creates the 'CrudFilters' object. See here to create a search form | Function | |
queryOptions | react-query 's useQuery options | UseQueryOptions<{ data: TData[] }, TError> | |
metaData | Metadata query for dataProvider | MetaDataQuery | {} |
liveMode | Whether to update data automatically ("auto" ) or not ("manual" ) if a related live event is received. The "off" value is used to avoid creating a subscription. | "auto" | "manual" | "off" | "off" |
liveParams | Params to pass to liveProvider 's subscribe method if liveMode is enabled. | { ids?: string[]; [key: string]: any; } | undefined |
onLiveEvent | Callback to handle all related live events of this hook. | (event: LiveEvent) => void | undefined |
Type Parameters
Property | Desription | Type | Default |
---|---|---|---|
TData | Result data of the mutation. Extends BaseRecord | BaseRecord | BaseRecord |
TError | Custom error object that extends HttpError | HttpError | HttpError |
TSearchVariables | Antd form values | {} | {} |
Return values
Property | Description | Type |
---|---|---|
queryResult | Result of the query of a record | QueryObserverResult<{ data: TData }> |
searchFormProps | Ant design Form props | Form |
listProps | Ant design List props | List |
filters | Current filters state | CrudFilters |