Import
<ImportButton>
is compatible with the useImport
hook and is meant to be used as it's upload button. It uses Material UI <LoadingButton>
component and native html <input>
element. It wraps a <label>
with a <LoadingButton>
component and <input>
element and accepts it's own properties for separately.
Refer to the for more detailed information about useImport
. →
Usage
Use it like any other Material UI <LoadingButton>
. You can use it with useImport:
/src/pages/posts/list.tsx
import { useTable, useImport } from "@pankod/refine-core";
import {
ImportButton,
List,
Table,
TableBody,
TableCell,
TableHead,
TableRow,
} from "@pankod/refine-mui";
export const PostList: React.FC = () => {
const { tableQueryResult } = useTable<IPost>();
const { inputProps, isLoading } = useImport<IPost>();
return (
<List
cardHeaderProps={{
action: (
<ImportButton inputProps={inputProps} loading={isLoading} />
),
}}
>
<Table>
<TableHead>
<TableRow>
<TableCell>ID</TableCell>
<TableCell>Title</TableCell>
</TableRow>
</TableHead>
<TableBody>
{tableQueryResult.data?.data.map((row) => (
<TableRow key={row.title}>
<TableCell>{row.id}</TableCell>
<TableCell component="th" scope="row">
{row.title}
</TableCell>
</TableRow>
))}
</TableBody>
</Table>
</List>
);
};
interface IPost {
id: number;
title: string;
}
It looks like this:
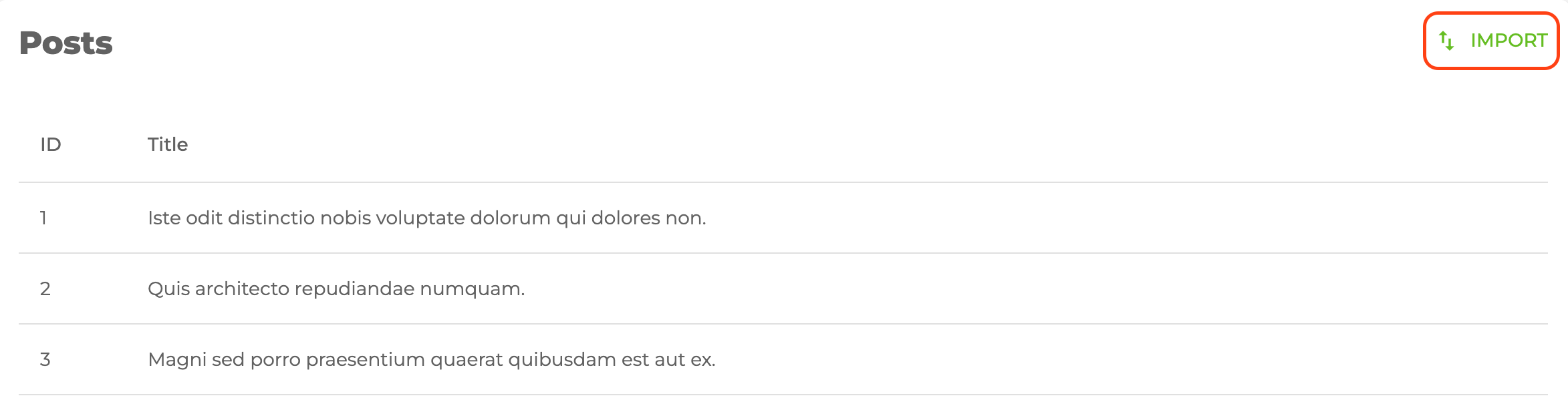
Properties
hideText
It is used to show and not show the text of the button. When true
, only the button icon is visible.
import { ImportButton } from "@pankod/refine-mui";
export const MyImportComponent = () => {
return <ImportButton hideText />;
};
API Reference
Properties
External Props
It also accepts all props of Material UI LoadingButton.