Date
This field is used to display dates. It uses Day.js
to display date format.
Usage
Let's see how we can use <DateField>
with the example in the post list.
src/pages/posts/list.tsx
import { useTable } from "@pankod/refine-core";
import {
Table,
TableBody,
TableCell,
TableHead,
TableRow,
List,
DateField,
} from "@pankod/refine-mui";
export const PostList: React.FC = () => {
const { tableQueryResult } = useTable<IPost>({
initialSorter: [
{
field: "id",
order: "asc",
},
],
});
const { data } = tableQueryResult;
return (
<List>
<Table aria-label="simple table">
<TableHead>
<TableRow>
<TableCell>Title</TableCell>
<TableCell align="center">Created At</TableCell>
</TableRow>
</TableHead>
<TableBody>
{data?.data.map((row) => (
<TableRow key={row.id}>
<TableCell component="th" scope="row">
{row.title}
</TableCell>
<TableCell align="center">
<DateField value={row.createdAt} />
</TableCell>
</TableRow>
))}
</TableBody>
</Table>
</List>
);
};
export interface IPost {
id: number;
title: string;
createdAt: string;
}
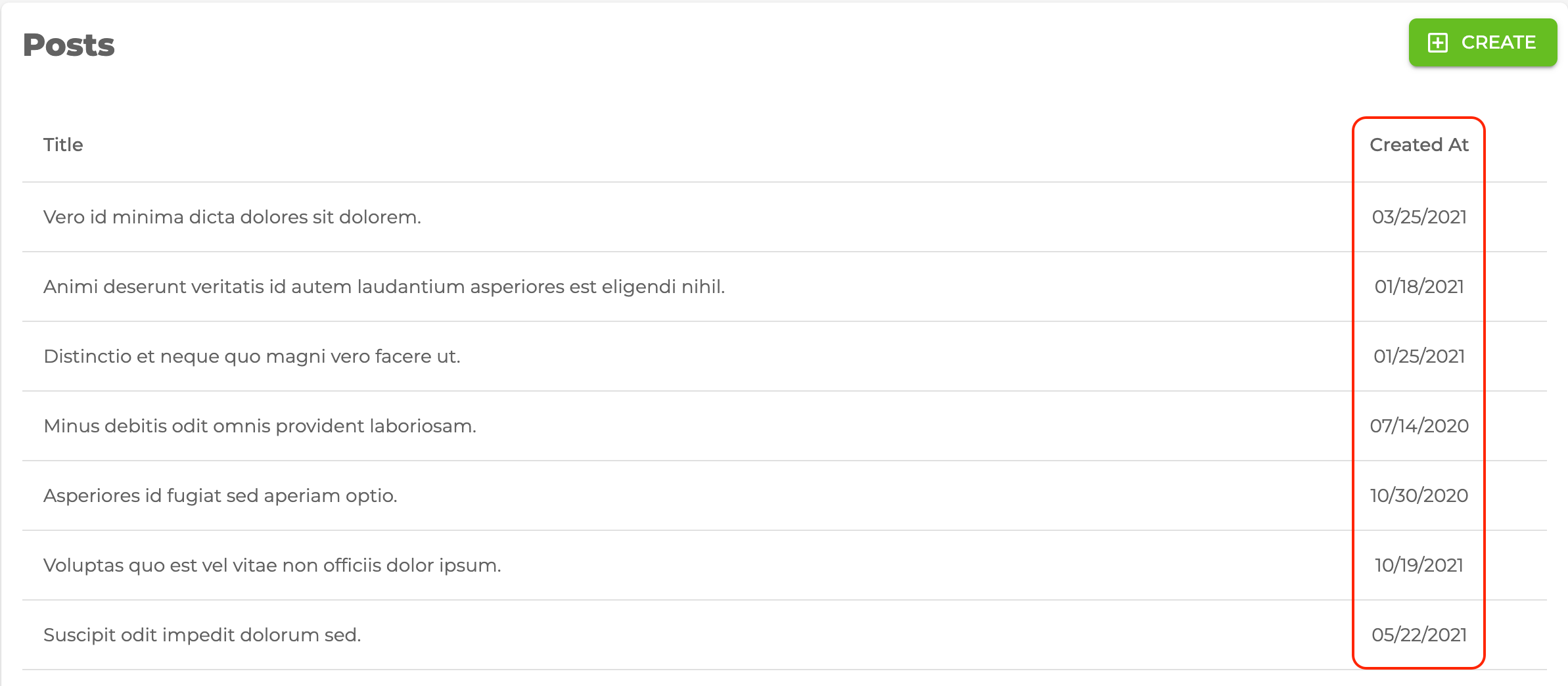
API Reference
Properties
External Props
It also accepts all props of Material UI Typography.